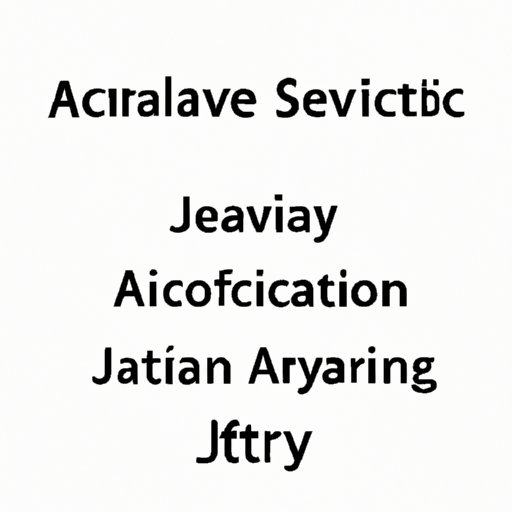
I. Introduction
If you’re just starting out in Java programming, you may be wondering how to declare arrays. Arrays are an important part of Java programming, as they allow you to store and manipulate multiple values in a single variable. However, declaring arrays correctly can be a bit tricky, especially if you’re new to programming. In this article, we’ll be exploring everything you need to know to declare arrays in Java, including best practices and common mistakes to avoid.
II. Understanding the Basics of Java Arrays: A Guide for Beginners
Before we dive into declaring arrays in Java, it’s helpful to understand what an array is and how it works. Simply put, an array is a data structure that allows you to store multiple values of the same data type in a single variable. This can be incredibly useful if you need to store and manipulate large sets of data.
In Java, arrays are declared using square brackets after the variable type. For example:
int[] myArray;
String[] myStringArray;
double[] myDoubleArray;
It’s important to note that declaring an array does not initialize it with any values. To initialize an array, you must use the new
keyword. For example:
int[] myArray = new int[5];
String[] myStringArray = new String[10];
double[] myDoubleArray = new double[3];
Here, we’re initializing three arrays with different sizes. The first array has a size of 5, the second has a size of 10, and the third has a size of 3.
III. 6 Ways to Declare Arrays in Java: Optimizing Your Code for Efficiency
There are several different ways to declare arrays in Java, each with its own benefits and drawbacks. Let’s take a look at some of the most common methods:
1. Declaring an Array of Primitive Data Types
The most basic way to declare an array in Java is to declare an array of primitive data types:
int[] myArray = new int[5];
double[] myDoubleArray = new double[3];
boolean[] myBooleanArray = new boolean[10];
This method is simple and straightforward, but it does have some limitations. For example, you can only store values of the same data type in the array. Additionally, you cannot resize the array once it has been created.
2. Declaring an Array of Objects
If you need to store objects in an array, you can declare an array of objects:
String[] myStringArray = new String[10];
Object[] myObjectArray = new Object[5];
Here, we’re declaring an array of strings and an array of objects. This method allows you to store objects of different types in the same array, but it can also be a bit more complex to work with than an array of primitive data types.
3. Declaring an Array Using Literals
If you know the values you want to store in an array ahead of time, you can declare the array using literals:
int[] myArray = {1, 2, 3, 4, 5};
String[] myStringArray = {"hello", "world", "java"};
double[] myDoubleArray = {1.0, 2.0, 3.0, 4.0, 5.0};
This method can be useful if you don’t need to resize the array, and it’s also a bit more concise than declaring an array using new
. However, it’s important to note that you cannot declare an empty array using literals.
4. Declaring an Array with a Specified Size
If you need to declare an array with a specific size but you don’t yet know what values you will store in it, you can declare an empty array with a specified size:
int[] myArray = new int[5];
String[] myStringArray = new String[10];
double[] myDoubleArray = new double[3];
This method is flexible and allows you to resize the array later if necessary. However, it does require more memory than declaring an array with an initial set of values.
5. Declaring an Array Without Specifying a Size
If you don’t yet know the size of the array you need, you can declare an array without specifying a size:
int[] myArray = new int[]{1, 2, 3, 4, 5};
String[] myStringArray = new String[]{"hello", "world", "java"};
double[] myDoubleArray = new double[]{1.0, 2.0, 3.0, 4.0, 5.0};
This method allows you to declare an array with an initial set of values without specifying the size. However, it can be less efficient than declaring an array with a specified size, as the JVM must allocate memory dynamically.
6. Declaring an Array Using the Enhanced For Loop
The enhanced for loop can also be used to declare arrays:
int[] myArray = {1, 2, 3, 4, 5};
for (int i : myArray) {
System.out.println(i);
}
This method is useful if you need to iterate through an array and perform an operation on each element. However, it’s important to note that you cannot initialize an array using the enhanced for loop.
IV. The Do’s and Don’ts of Declaring Arrays in Java: Best Practices for Success
Now that we’ve explored some of the different ways to declare arrays in Java, let’s take a look at some best practices for declaring arrays:
1. Declare an Array with the Appropriate Size
When declaring an array, it’s important to declare it with the appropriate size. If your array is too small, you may not be able to store all the data you need. If it’s too large, you’re wasting memory. Take the time to consider how many elements you need to store in your array and declare it with the appropriate size.
2. Use Meaningful Variable Names
When declaring arrays, it’s important to use meaningful variable names that accurately describe the contents of the array. This can make your code easier to understand and maintain.
3. Initialize Arrays
Always initialize arrays when declaring them. This ensures that all elements in the array are set to a default value, which can prevent unexpected behavior in your code.
4. Avoid Magic Numbers
When declaring arrays, avoid using “magic numbers” or hardcoded values. Instead, use constants or variables to represent these values. This can make your code more flexible and easier to maintain.
5. Keep Arrays as Small as Possible
Try to keep arrays as small as possible. Larger arrays use more memory, which can impact the performance of your code. Consider using other data structures, such as lists or maps, if they better suit your needs.
6. Avoid Redundant Code
Avoid declaring redundant arrays that store identical data. This can use up unnecessary memory and slow down your program. Instead, use methods to manipulate existing arrays.
7. Understand the Scope of Arrays
Be aware of the scope of your arrays. If you declare an array inside a loop, for example, it will only be accessible within that loop. Ensure that your arrays are declared in the appropriate scope for your needs.
V. 3 Simple Steps to Declaring Arrays in Java: A Quick and Easy Tutorial
If you’re looking for a quick and easy tutorial on declaring arrays in Java, you’re in luck. Follow these three simple steps:
Step 1: Declare the Array Variable
Declare the array variable using the appropriate syntax:
int[] myArray;
Step 2: Initialize the Array
Initialize the array using the new
keyword:
myArray = new int[5];
Step 3: Assign Values to the Array
Assign values to the array using the appropriate syntax:
myArray[0] = 1;
myArray[1] = 2;
myArray[2] = 3;
myArray[3] = 4;
myArray[4] = 5;
And that’s it! You’ve successfully declared and initialized an array in Java.
VI. From Single-Dimensional to Multi-Dimensional: A Comprehensive Guide to Java Arrays
So far, we’ve only looked at single-dimensional arrays. However, it’s also possible to declare multi-dimensional arrays in Java. A multi-dimensional array is simply an array of arrays.
To declare a multi-dimensional array, use the following syntax:
int[][] myArray = new int[3][3];
This creates a 3×3 array of integers. You can also initialize a multi-dimensional array using literals:
int[][] myArray = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
This creates the same 3×3 array of integers, but it initializes it with values.
VII. Common Mistakes to Avoid When Declaring Arrays in Java: Tips for Better Programming
Now that we’ve explored best practices for declaring arrays, let’s take a look at some common mistakes to avoid:
1. Declaring an Array with the Wrong Data Type
Make sure that you declare your array with the correct data type. If you declare an array with the wrong data type, you may get unexpected results or errors.
2. Forgetting to Initialize an Array
Always initialize your arrays when declaring them. Forgetting to initialize an array can lead to unexpected behavior in your code.
3. Trying to Access Elements Outside the Bounds of an Array
Be careful when accessing elements in an array. Trying to access an element outside the bounds of an array can result in an ArrayIndexOutOfBoundsException.
VIII. Conclusion
Declaring arrays in Java can seem daunting at first, but with practice and the right techniques, it can become second nature. This article has covered everything from the basics of Java arrays to best practices for success. We hope that you now feel confident in your ability to declare and use arrays in your Java programs.
Remember to always declare arrays with the appropriate size, use meaningful variable names, and initialize your arrays. Avoid common mistakes such as declaring arrays with the wrong data type and trying to access elements outside the bounds of an array.